大学c语言考试编程题
Title: Challenging C Programming Exercises for University Students
1.
Palindrome Checker:
Write a C program to check if a given string is a palindrome or not. A palindrome is a string that reads the same backward as forward.
```c
include
include
int main() {
char str[100];
int i, len, flag = 0;
printf("Enter a string: ");
scanf("%s", str);
len = strlen(str);
for(i = 0; i < len/2; i ) {
if(str[i] != str[leni1]) {
flag = 1;
break;
}
}
if(flag)
printf("%s is not a palindrome.\n", str);
else
printf("%s is a palindrome.\n", str);
return 0;
}
```
2.
Prime Number Generator:
Develop a C program to generate prime numbers up to a userspecified limit using the Sieve of Eratosthenes algorithm.
```c
include
include
define MAX_LIMIT 1000
int main() {
int limit, i, j;
bool isPrime[MAX_LIMIT];
printf("Enter the limit: ");
scanf("%d", &limit);
// Assume all numbers are prime initially
for (i = 2; i <= limit; i )
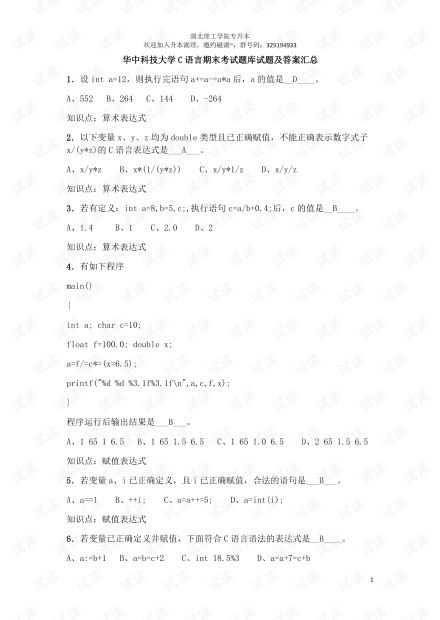
isPrime[i] = true;
// Apply Sieve of Eratosthenes algorithm
for (i = 2; i * i <= limit; i ) {
if (isPrime[i]) {
for (j = i * i; j <= limit; j = i)
isPrime[j] = false;
}
}
// Print prime numbers
printf("Prime numbers up to %d are: ", limit);
for (i = 2; i <= limit; i ) {
if (isPrime[i])
printf("%d ", i);
}
printf("\n");
return 0;
}
```
3.
Linked List Operations:
Implement basic operations on a singly linked list such as insertion, deletion, and traversal.
```c
include
include
struct Node {
int data;
struct Node* next;
};
// Function to insert a node at the beginning of a linked list
void insertAtBeginning(struct Node** head_ref, int new_data) {
struct Node* new_node = (struct Node*)malloc(sizeof(struct Node));
new_node>data = new_data;
new_node>next = *head_ref;
*head_ref = new_node;
}
// Function to delete a node with a given key
void deleteNode(struct Node** head_ref, int key) {
struct Node* temp = *head_ref, *prev;
if (temp != NULL && temp>data == key) {
*head_ref = temp>next;
free(temp);
return;
}
while (temp != NULL && temp>data != key) {
prev = temp;
temp = temp>next;
}
if (temp == NULL)
return;
prev>next = temp>next;
free(temp);
}
// Function to print the elements of a linked list
void printList(struct Node* node) {
while (node != NULL) {
printf("%d ", node>data);
node = node>next;
}
printf("\n");
}
int main() {
struct Node* head = NULL;
insertAtBeginning(&head, 5);
insertAtBeginning(&head, 4);
insertAtBeginning(&head, 3);
insertAtBeginning(&head, 2);
insertAtBeginning(&head, 1);
printf("Linked list: ");
printList(head);
deleteNode(&head, 3);
printf("Linked list after deleting 3: ");
printList(head);
return 0;
}
```
These exercises cover a range of topics in C programming, including string manipulation, algorithm implementation, and data structures. They are designed to challenge university students and enhance their understanding of the language.
评论