Title: Building a Code System: Best Practices and Considerations
Creating a robust and efficient code system is essential for any programming project, whether it's a smallscale application or a largescale enterprise software. A welldesigned code system not only ensures the functionality of the software but also facilitates maintenance, scalability, and collaboration among developers. Let's delve into the key considerations and best practices for building a code system.
1. Modular Design:
Break down the system into modular components, each responsible for specific functionality.
Encapsulate related functionalities within modules to promote code reusability and maintainability.
Utilize techniques like objectoriented programming or functional programming paradigms to implement modular design.
2. Clear Documentation:
Document each module, class, function, and method with clear descriptions of their purpose, inputs, outputs, and usage examples.
Use documentation tools like Javadoc, Doxygen, or Sphinx to generate comprehensive documentation automatically.
Maintain updated documentation to aid developers in understanding and using the codebase effectively.
3. Consistent Coding Style:
Establish a coding style guide outlining conventions for formatting, naming conventions, and code structure.
Enforce the coding style through code reviews, automated linting tools, and continuous integration pipelines.
Consistency in coding style enhances readability and reduces cognitive overhead when navigating the codebase.
4. Version Control:
Utilize a version control system such as Git to track changes, collaborate with team members, and manage codebase history.
Follow branching strategies like GitFlow or GitHub Flow to organize development, testing, and deployment workflows.
Regularly commit changes with descriptive commit messages to provide context and facilitate code review processes.
5. Testing Strategy:
Implement a comprehensive testing strategy encompassing unit tests, integration tests, and endtoend tests.
Automate testing processes using frameworks like JUnit, Pytest, or Selenium to ensure consistent and reliable test execution.
Adopt testdriven development (TDD) practices to write tests before implementing code, promoting test coverage and code quality.
6. Error Handling and Logging:
Implement robust error handling mechanisms to gracefully handle unexpected exceptions and errors.
Utilize logging frameworks like Log4j, Logback, or Python's logging module to record informative logs for debugging and monitoring purposes.
Configure log levels appropriately to balance verbosity and relevance of log messages.
7. Performance Optimization:
Profile code performance using profiling tools to identify bottlenecks and areas for optimization.
Employ techniques like caching, algorithmic improvements, and resource optimization to enhance system performance.
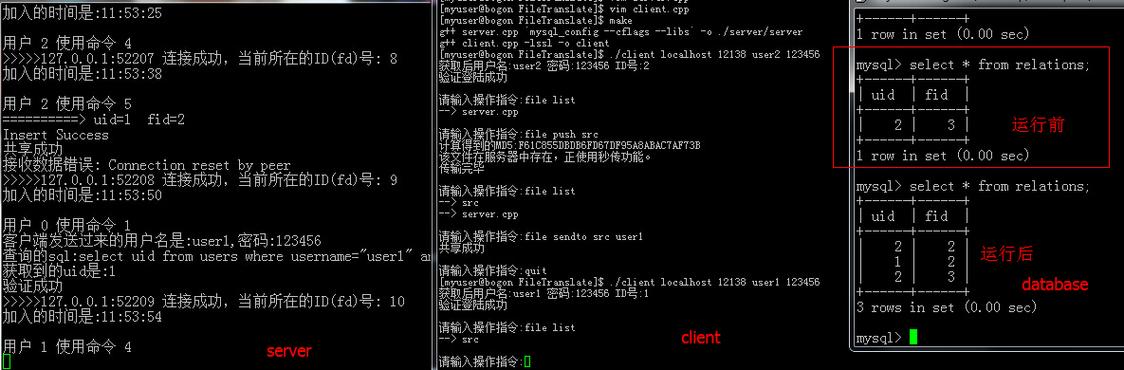
Continuously monitor and benchmark performance metrics to ensure optimal system efficiency.
8. Security Measures:
Implement security best practices such as input validation, authentication, and authorization mechanisms.
Sanitize user inputs to prevent injection attacks and mitigate security vulnerabilities.
Stay updated with security patches and vulnerabilities in dependencies to maintain a secure codebase.
9. Scalability and Flexibility:
Design the code system with scalability in mind to accommodate future growth and evolving requirements.
Utilize scalable architectures like microservices, serverless computing, or containerization to facilitate horizontal scaling.
Decouple components and minimize dependencies to enhance flexibility and modifiability.
10. Continuous Integration and Deployment (CI/CD):
Automate build, test, and deployment processes using CI/CD pipelines to streamline development workflows.
Integrate with tools like Jenkins, Travis CI, or GitLab CI for automated testing and deployment.
Ensure continuous integration of code changes to detect issues early and deliver updates frequently and reliably.
In conclusion, building a code system requires careful planning, adherence to best practices, and continuous refinement. By following these guidelines and incorporating industry standards and emerging technologies, developers can create robust, maintainable, and scalable code systems that meet the demands of modern software development.
评论