vs2019多线程调试
Title: Guide to Multithreading Programming in Visual Studio 2012
Multithreading programming in Visual Studio 2012 involves leveraging the power of concurrency to enhance application performance and responsiveness. Here's a comprehensive guide to help you navigate through the intricacies of multithreading in Visual Studio 2012:
Understanding Multithreading:
Multithreading is the ability of a CPU (Central Processing Unit) to provide multiple threads of execution concurrently. In simpler terms, it allows a program to perform multiple tasks simultaneously, thereby maximizing CPU utilization and improving overall efficiency.
Creating Threads in Visual Studio 2012:
In Visual Studio 2012, you can create threads using various techniques, including:
1.
Thread Class
: Utilize the `System.Threading.Thread` class to create and manage threads. You can instantiate a new thread by passing a delegate to the thread's constructor, specifying the method to be executed by the thread.```csharp
Thread myThread = new Thread(MyMethod);
myThread.Start();
```
2.
ThreadPool
: Employ the .NET ThreadPool to efficiently manage and reuse threads for executing asynchronous tasks. This approach is beneficial for scenarios involving numerous shortlived tasks.```csharp
ThreadPool.QueueUserWorkItem(MyMethod);
```
3.
Task Parallel Library (TPL)
: TPL simplifies multithreading by abstracting the underlying thread management. Use the `Task` class to represent asynchronous operations, allowing for easier composition of parallel tasks.```csharp
Task.Run(() => MyMethod());
```
Synchronization and Thread Safety:
Concurrency introduces the risk of race conditions and data inconsistencies when multiple threads access shared resources simultaneously. Visual Studio 2012 provides synchronization mechanisms to ensure thread safety:
1.
Locking
: Employ the `lock` keyword to create mutually exclusive sections of code, preventing concurrent access to shared resources.```csharp
lock (lockObject)
{
// Access shared resource safely
}
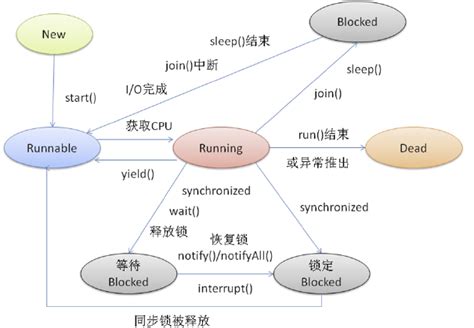
```
2.
Monitor Class
: Use the `Monitor` class for more finegrained control over synchronization. It provides methods like `Enter()` and `Exit()` to acquire and release locks explicitly.```csharp
Monitor.Enter(lockObject);
try
{
// Access shared resource safely
}
finally
{
Monitor.Exit(lockObject);
}
```
3.
Mutex and Semaphore
: For interprocess synchronization, Visual Studio 2012 offers synchronization primitives like `Mutex` and `Semaphore`, which enable coordination across multiple processes.Avoiding Deadlocks:
Deadlocks occur when two or more threads are blocked indefinitely, waiting for each other to release resources. To prevent deadlocks:
1.
Lock Ordering
: Establish a consistent order for acquiring locks across threads to avoid circular dependencies.2.
Timeouts
: Implement timeouts when acquiring locks to prevent threads from waiting indefinitely.3.
Avoid Nested Locks
: Minimize the scope of locked regions and avoid nested locks to reduce the likelihood of deadlocks.Debugging Multithreaded Applications:
Debugging multithreaded applications can be challenging due to nondeterministic behavior. Visual Studio 2012 provides powerful debugging tools to aid in identifying and resolving concurrency issues:
1.
Parallel Stacks
: Use Parallel Stacks window to visualize the call stack of each thread, facilitating identification of threadrelated issues.2.
Thread Window
: Monitor the state of individual threads, including their call stacks and execution status.3.
Thread Profiling
: Utilize performance profiling tools to identify bottlenecks and optimize multithreaded code for improved efficiency.Best Practices for Multithreading in Visual Studio 2012:
To maximize the benefits of multithreading and ensure robustness of your applications, adhere to the following best practices:
1.
Minimize Shared State
: Reduce reliance on shared resources to minimize the risk of contention and simplify synchronization.2.
Use Immutable Data
: Immutable data structures eliminate the need for synchronization by ensuring that data cannot be modified after creation.3.
Asynchronous Programming
: Embrace asynchronous programming patterns using async/await keywords to improve responsiveness without blocking threads.4.
Testing and Validation
: Thoroughly test multithreaded code under various scenarios to identify and rectify concurrency issues before deployment.By following these guidelines and leveraging the capabilities of Visual Studio 2012, you can effectively harness the power of multithreading to build scalable and responsive applications.
Conclusion:
Multithreading programming in Visual Studio 2012 offers immense potential for enhancing application performance and responsiveness. By understanding the fundamentals of concurrency, employing appropriate synchronization mechanisms, and leveraging debugging tools, you can develop robust and efficient multithreaded applications. Remember to adhere to best practices and continually optimize your code to ensure optimal performance and reliability.
评论