程序设计模式有几种
Title: Understanding JavaScript's indexOf Method
JavaScript's `indexOf` method is a powerful tool for searching arrays and strings. Whether you're a beginner or an experienced developer, mastering this method is essential for efficient and effective programming. In this guide, we'll delve into the intricacies of `indexOf`, covering its syntax, applications, and best practices.
Syntax:
The `indexOf` method is used to search for a specified item in an array or a string and returns the index of the first occurrence of the item.
For Arrays:
```javascript
array.indexOf(searchElement[, fromIndex])
```
For Strings:
```javascript
string.indexOf(searchValue[, fromIndex])
```
`searchElement` or `searchValue`: The value to search for.
`fromIndex` (optional): The index to start the search from. If not specified, the search starts from index 0.
Return Value:
If the element or value is found, `indexOf` returns the index of the first occurrence.
If not found, it returns 1.
Examples:
Searching in Arrays:
```javascript
const fruits = ['apple', 'banana', 'orange', 'apple'];
console.log(fruits.indexOf('apple')); // Output: 0
console.log(fruits.indexOf('banana')); // Output: 1
console.log(fruits.indexOf('pear')); // Output: 1
```
Searching in Strings:
```javascript
const sentence = 'JavaScript is amazing!';
console.log(sentence.indexOf('is')); // Output: 11
console.log(sentence.indexOf('Python')); // Output: 1
```
Tips and Best Practices:
1.
Check for Presence:
Always check the return value of `indexOf` against 1 to ensure that the searched item exists in the array or string.
```javascript
if (array.indexOf('item') !== 1) {
// Item found
} else {
// Item not found
}
```
2.
Searching for All Occurrences:
If you need to find all occurrences of an item, consider using a loop or combining `indexOf` with a loop or other array methods.
```javascript
const indices = [];
let idx = array.indexOf('item');
while (idx !== 1) {
indices.push(idx);
idx = array.indexOf('item', idx 1);
}
```
3.
Avoiding Type Coercion Issues:
Be cautious when using `indexOf` with loose equality (==) comparisons, as it may lead to unexpected results due to type coercion.
```javascript
const array = [1, 2, 3, 10];
console.log(array.indexOf('1')); // Output: 1 (expected result might be 0)
console.log(array.indexOf(1)); // Output: 0
```
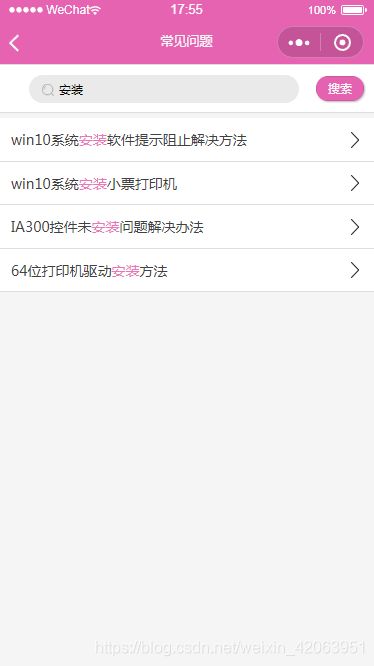
4.
Consider Compatibility:
`indexOf` is supported in all modern browsers, but if you need to support older browsers, consider using polyfills or alternative methods like `Array.prototype.includes`.
Conclusion:
JavaScript's `indexOf` method is a versatile tool for searching arrays and strings. By understanding its syntax, return values, and best practices, you can leverage its power to write cleaner, more efficient code. Remember to handle edge cases and consider compatibility when using this method in your projects. With practice, you'll master `indexOf` and enhance your JavaScript programming skills.
This guide serves as a comprehensive resource for both beginners and experienced developers looking to deepen their understanding of JavaScript's `indexOf` method.
评论