shell编程阶乘
Title: Mastering Shell Scripting for Calculating Products
```html
Mastering Shell Scripting for Calculating Products
Shell scripting is a powerful tool for automating tasks and processing data in Unixbased systems. When it comes to calculating products within a shell script, there are several approaches you can take. Let's explore some methods:
This method involves using a loop to iterate through the numbers and calculate their product.
```bash
!/bin/bash
Initialize the product variable
product=1
Numbers to multiply
numbers=(2 4 6 8 10)
Loop through the numbers and calculate the product
for num in "${numbers[@]}"; do
((product *= num))
done
echo "The product of ${numbers[@]} is: $product"
```
This script initializes a variable called 'product' to 1. Then it iterates through an array of numbers, multiplying each number with the 'product' variable. Finally, it prints out the result.
Another approach is to pass the numbers as commandline arguments to the script.
```bash
!/bin/bash
Check if no arguments are passed
if [ $ eq 0 ]; then
echo "Usage: $0
exit 1
fi
Initialize the product variable
product=1
Loop through the arguments and calculate the product
for num in "$@"; do
((product *= num))
done
echo "The product of $@ is: $product"
```
This script checks if any arguments are passed. If not, it displays usage instructions. Otherwise, it initializes the 'product' variable to 1 and multiplies each argument to calculate the product.
If dealing with floatingpoint numbers, you can use the 'bc' command for precision arithmetic.
```bash
!/bin/bash
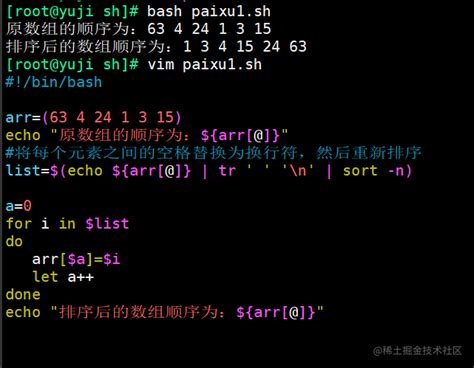
Numbers to multiply
numbers="2.5 3.5 4.5"
Calculate the product using bc command
product=$(echo "$numbers" | tr ' ' '*' | bc)
echo "The product of $numbers is: $product"
```
This script uses the 'bc' command to perform the multiplication. It first replaces spaces between numbers with '*' using 'tr' command and then passes it to 'bc' for calculation.
Shell scripting provides various methods for calculating products based on your requirements. Whether it's through loops, commandline arguments, or utilizing external commands like 'bc', you can efficiently compute products within shell scripts.